Getting Started Plugins
Overview
The Payment Module in the Exsited SDK provides a robust set of tools to handle payment-related operations within your application. It allows developers to streamline payment processing, apply payments to invoices, and retrieve detailed payment histories for auditing or reconciliation purposes. Designed for versatility, this module supports a wide range of payment processors and ensures accurate financial tracking.
Function: createBasicPayment
Purpose
The createBasicPayment()
function creates a payment for a specified invoice by submitting payment details, including the payment processor, amount, and reference. It also includes additional details such as the payment date and associated notes. The function validates the creation of the payment and returns the payment details upon success.
Parameters
Parameter | Type | Description |
---|---|---|
invoice_id | String | The unique identifier for the invoice associated with the payment. |
date | String (Date) | The date when the payment was made (format: YYYY-MM-DD). |
note | String | A note or description related to the payment. |
processor | String | The processor or method of payment (e.g., "Cash", "Credit"). |
amount | String (Decimal) | The amount of money applied in the payment (e.g., "20.00"). |
reference | String | A reference or identifier for the payment transaction. |
Function: readInvoicePaymentDetails
Purpose
The readInvoicePaymentDetails()
function retrieves detailed payment information for a specified invoice. This function is useful for reviewing payment transactions, reconciling records, and ensuring the correct application of payments to the invoice.
Parameters
Parameter | Type | Description |
---|---|---|
id
|
String | The unique identifier of the invoice for which payment details are being retrieved. |
Use Case
This function is used when detailed payment information is required for auditing, customer queries, or verifying payment records against a specific invoice. For example, it can be applied in financial systems to review applied payments and their statuses.
PaymentInvoiceResponseDTO(
invoice=PaymentListDTO(
payments=[
PaymentDataDTO(
id='PAYMENT_ID_1',
date='PAYMENT_DATE_1',
status='PAYMENT_STATUS_1',
reconcileStatus='RECONCILE_STATUS_1',
totalApplied='TOTAL_APPLIED_1',
paymentApplied=[
PaymentAppliedDTO(
processor='PROCESSOR_1',
amount=AMOUNT_1,
reference='REFERENCE_1',
method='METHOD_1'
)
],
note='NOTE_1',
creditApplied=[],
invoices=[
InvoiceDTO(
applied='APPLIED_AMOUNT_1',
code='INVOICE_CODE_1',
dueDate='DUE_DATE_1',
issueDate='ISSUE_DATE_1',
outstanding='OUTSTANDING_AMOUNT_1',
total='TOTAL_AMOUNT_1'
)
],
createdBy='CREATED_BY_1',
createdOn='CREATED_ON_1',
lastUpdatedBy='LAST_UPDATED_BY_1',
lastUpdatedOn='LAST_UPDATED_ON_1',
uuid='UUID_1',
version='VERSION_1',
customAttributes=[],
customObjects=[],
giftCertificateApplied=[]
),
PaymentDataDTO(
id='PAYMENT_ID_2',
date='PAYMENT_DATE_2',
status='PAYMENT_STATUS_2',
reconcileStatus='RECONCILE_STATUS_2',
totalApplied='TOTAL_APPLIED_2',
paymentApplied=[
PaymentAppliedDTO(
processor='PROCESSOR_2',
amount=AMOUNT_2,
reference='REFERENCE_2',
method='METHOD_2'
)
],
note='NOTE_2',
creditApplied=[],
invoices=[
InvoiceDTO(
applied='APPLIED_AMOUNT_2',
code='INVOICE_CODE_2',
dueDate='DUE_DATE_2',
issueDate='ISSUE_DATE_2',
outstanding='OUTSTANDING_AMOUNT_2',
total='TOTAL_AMOUNT_2'
)
],
createdBy='CREATED_BY_2',
createdOn='CREATED_ON_2',
lastUpdatedBy='LAST_UPDATED_BY_2',
lastUpdatedOn='LAST_UPDATED_ON_2',
uuid='UUID_2',
version='VERSION_2',
customAttributes=[],
customObjects=[],
giftCertificateApplied=[]
),
PaymentDataDTO(
id='PAYMENT_ID_3',
date='PAYMENT_DATE_3',
status='PAYMENT_STATUS_3',
reconcileStatus='RECONCILE_STATUS_3',
totalApplied='TOTAL_APPLIED_3',
paymentApplied=[
PaymentAppliedDTO(
processor='PROCESSOR_3',
amount=AMOUNT_3,
reference='REFERENCE_3',
method='METHOD_3'
)
],
note='NOTE_3',
creditApplied=[],
invoices=[
InvoiceDTO(
applied='APPLIED_AMOUNT_3',
code='INVOICE_CODE_3',
dueDate='DUE_DATE_3',
issueDate='ISSUE_DATE_3',
outstanding='OUTSTANDING_AMOUNT_3',
total='TOTAL_AMOUNT_3'
)
],
createdBy='CREATED_BY_3',
createdOn='CREATED_ON_3',
lastUpdatedBy='LAST_UPDATED_BY_3',
lastUpdatedOn='LAST_UPDATED_ON_3',
uuid='UUID_3',
version='VERSION_3',
customAttributes=[],
customObjects=[],
giftCertificateApplied=[]
)
],
pagination=PaginationDTO(
records='TOTAL_RECORDS',
limit='LIMIT',
offset='OFFSET',
previousPage='PREVIOUS_PAGE',
nextPage='NEXT_PAGE'
)
)
)
{
"invoice": {
"payments": [
{
"status": "PAYMENT_STATUS_1",
"id": "PAYMENT_ID_1",
"date": "PAYMENT_DATE_1",
"reconcile_status": "RECONCILE_STATUS_1",
"total_applied": "TOTAL_APPLIED_1",
"payment_applied": [
{
"amount": "AMOUNT_1",
"method": "METHOD_1",
"processor": "PROCESSOR_1",
"reference": "REFERENCE_1"
}
],
"credit_applied": [],
"gift_certificate_applied": [],
"invoices": [
{
"applied": "APPLIED_AMOUNT_1",
"code": "INVOICE_CODE_1",
"due_date": "DUE_DATE_1",
"issue_date": "ISSUE_DATE_1",
"outstanding": "OUTSTANDING_AMOUNT_1",
"total": "TOTAL_AMOUNT_1"
}
],
"created_by": "CREATED_BY_1",
"created_on": "CREATED_ON_1",
"last_updated_by": "LAST_UPDATED_BY_1",
"last_updated_on": "LAST_UPDATED_ON_1",
"uuid": "UUID_1",
"version": "VERSION_1",
"custom_attributes": [],
"custom_objects": []
},
{
"status": "PAYMENT_STATUS_2",
"id": "PAYMENT_ID_2",
"date": "PAYMENT_DATE_2",
"reconcile_status": "RECONCILE_STATUS_2",
"total_applied": "TOTAL_APPLIED_2",
"payment_applied": [
{
"amount": "AMOUNT_2",
"method": "METHOD_2",
"processor": "PROCESSOR_2",
"reference": "REFERENCE_2"
}
],
"credit_applied": [],
"gift_certificate_applied": [],
"invoices": [
{
"applied": "APPLIED_AMOUNT_2",
"code": "INVOICE_CODE_2",
"due_date": "DUE_DATE_2",
"issue_date": "ISSUE_DATE_2",
"outstanding": "OUTSTANDING_AMOUNT_2",
"total": "TOTAL_AMOUNT_2"
}
],
"created_by": "CREATED_BY_2",
"created_on": "CREATED_ON_2",
"last_updated_by": "LAST_UPDATED_BY_2",
"last_updated_on": "LAST_UPDATED_ON_2",
"uuid": "UUID_2",
"version": "VERSION_2",
"custom_attributes": [],
"custom_objects": []
},
{
"status": "PAYMENT_STATUS_3",
"id": "PAYMENT_ID_3",
"date": "PAYMENT_DATE_3",
"reconcile_status": "RECONCILE_STATUS_3",
"total_applied": "TOTAL_APPLIED_3",
"payment_applied": [
{
"amount": "AMOUNT_3",
"method": "METHOD_3",
"processor": "PROCESSOR_3",
"reference": "REFERENCE_3"
}
],
"credit_applied": [],
"gift_certificate_applied": [],
"invoices": [
{
"applied": "APPLIED_AMOUNT_3",
"code": "INVOICE_CODE_3",
"due_date": "DUE_DATE_3",
"issue_date": "ISSUE_DATE_3",
"outstanding": "OUTSTANDING_AMOUNT_3",
"total": "TOTAL_AMOUNT_3"
}
],
"created_by": "CREATED_BY_3",
"created_on": "CREATED_ON_3",
"last_updated_by": "LAST_UPDATED_BY_3",
"last_updated_on": "LAST_UPDATED_ON_3",
"uuid": "UUID_3",
"version": "VERSION_3",
"custom_attributes": [],
"custom_objects": []
}
],
"pagination": {
"records": "TOTAL_RECORDS",
"limit": "LIMIT",
"offset": "OFFSET",
"previous_page": "PREVIOUS_PAGE",
"next_page": "NEXT_PAGE"
}
}
}
Language-Specific Features
Feature |
Python Implementation |
PHP Implementation |
---|---|---|
SDK Initialization |
ExsitedSDK().init_sdk() |
$this->paymentManager |
Exception Handling |
ABException handles errors and raw response |
try-catch block for exceptions |
Response Format |
Prints response using print() |
Outputs response using json_encode() |
Configuration |
Managed through SDKConfig |
Managed via ConfigManager |
Authentication Method |
OAuth token via CommonData.get_request_token_dto() |
API credentials via $this->paymentManager |
Error Logging |
Captures errors with ABException and prints raw response |
Captures exceptions and prints error message |
Data Parsing |
JSON response parsed natively in Python |
JSON encoded and displayed using PHP |
Error Output |
print(ab.get_errors()) and ab.raw_response |
echo 'Error: ' . $e->getMessage() |
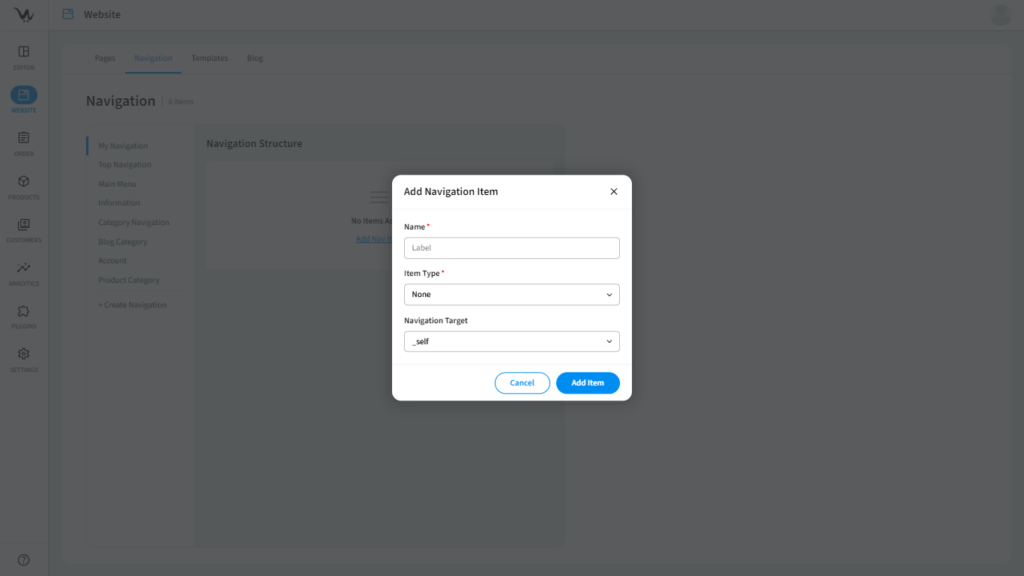